协作式AI助手
协作式AI助手,可嵌入系统页面中进行智能信息查询
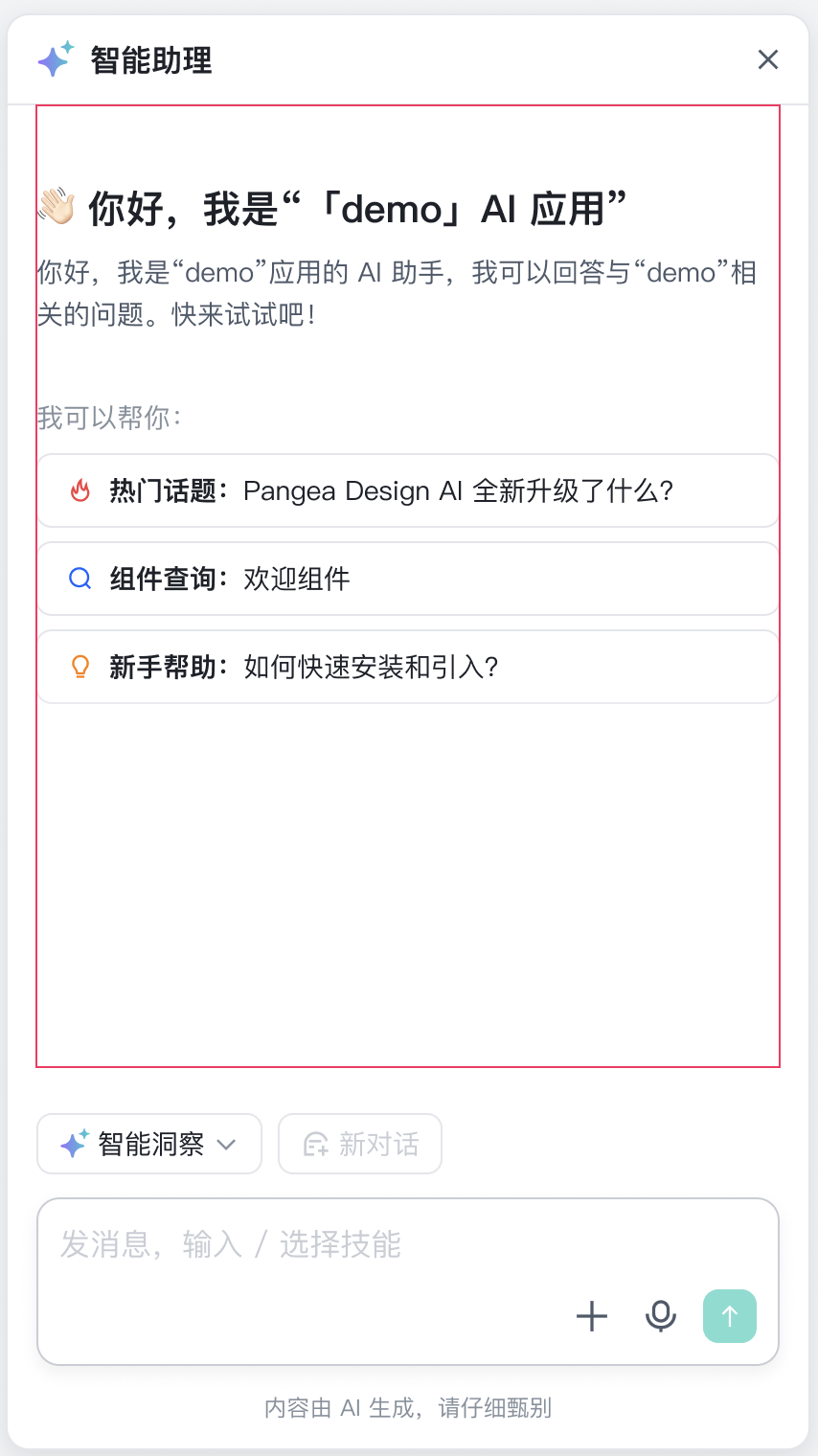
安装方式
bash
yarn add pangea-component
引用方式
js
import HiChatModal from "pangea-component/hi-chat-modal";
import "pangea-component/dist/style.css";
API
Props
参数名 | 描述 | 类型 | 默认值 |
---|---|---|---|
messages | 聊天列表数据 | Message[] | [] |
loading | 聊天问题是否正在查询中(是否显示停止生成操作) | Boolean | false |
title | 标题 | string | - |
description | 描述 | string | - |
suggestions | 建议问题列表 | SuggestionItem[] | [] |
javascript
interface Message {
type: "ai" | "user"; // 消息类型
id: number;
content: string; // 消息内容
thougth?: string; // 思考内容
pageJson?: any; // 页面json
isAgentThought?: boolean; // 是否具有思考内容
isThoughtCollapsed?: boolean; // 是否折叠思考内容
status?: "loading" | "success" | "fail"; // 消息状态
}
interface SuggestionItem {
title: string; // 主标题
subTitle?: string; // 子标题
icon?: VNode; // 图标
}
Events
方法名 | 描述 | 参数 |
---|---|---|
send | 输入框发送事件 | (value: string) => {} |
stop | 打断当前发送事件 | () => {} |
newChat | 新建对话事件 | () => {} |
Slot
插槽名 | 描述 | 参数 |
---|---|---|
sender-top | 输入框顶部插槽 | - |
sender-prefix | 输入框底部左侧扩展插槽 | - |
welcome | 欢迎区域插槽 | - |
Methods
方法名 | 描述 | 参数 | 返回值 |
---|---|---|---|
open | 打开ai助手 | - | - |
close | 关闭ai助手 | - | - |
scrollToBottom | 会话信息列表内容滚动到底部方法 | - | - |
使用示例
html
<template>
<button @click="showAiAssistant">Ai助手</button>
<hi-chat-modal
ref="hiChatRef"
:messages="messages"
:loading="loading"
:suggestions="suggestions"
:title="agentInfo.name"
:description="agentConfig.opening_statement"
@send="send"
@stop="stop"
@newChat="newChat"
>
</hi-chat-modal>
</template>
<script lang="ts" setup>
import { ref, onMounted, computed } from "vue";
import HiChatModal from "pangea-component/hi-chat-modal";
import useChat from "pangea-component/use-chat";
const hiChatRef = ref();
const {
sendChat,
stopChat,
messages,
loading,
conversationId,
queryAgentConfig,
queryAgentInfo,
agentInfo,
agentConfig,
} = useChat({ chatRef: hiChatRef });
const suggestions = computed(() => {
if (agentConfig.value?.suggested_questions?.length) {
return agentConfig.value.suggested_questions.map((item) => {
return {
subTitle: item,
};
});
}
return [];
});
onMounted(() => {
queryAgentConfig();
queryAgentInfo();
});
const send = (value) => {
sendChat(value);
};
const stop = () => {
stopChat();
};
const newChat = () => {
messages.value = [];
conversationId.value = null;
};
const showAiAssistant = () => {
hiChatRef.value.open();
};
</script>
<style lang="less" scoped></style>