流程审批移动端
流程审批组件用于流程任务的审批,用户可以查阅任务信息和流程操作记录,通过审批组件完成流程表单的录入,并执行各种自定义操作。组件支持定义和执行自定义的流程处理逻辑,确保审批流程的灵活性和高效性。
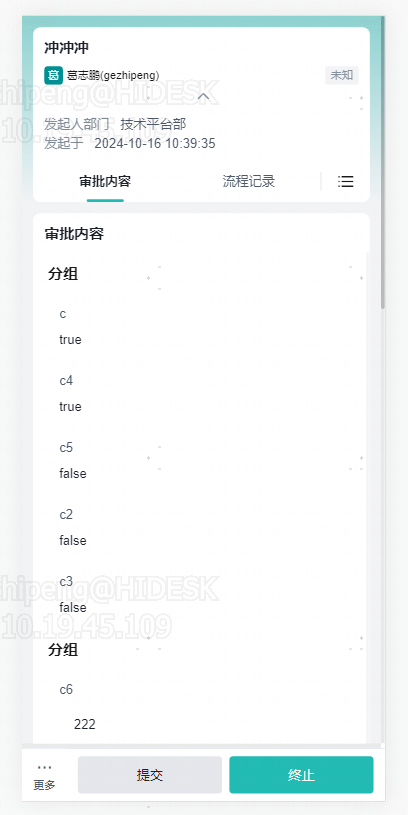
安装方式
bash
yarn add pangea-component-mobile
引用方式
js
import HiFlowApproval from "pangea-component-mobile/hi-m-flow-approval";
API
Props
参数名 | 描述 | 类型 | 默认值 |
---|---|---|---|
data | 审批基本信息 | Data | {} |
operations | 操作按钮列表 | OptionItem[] | [] |
operateRecords | 流程审批记录 | OperateRecord[] | [] |
circulateRecords | 流程传阅记录 | CirculateRecord[] | [] |
approvalConfig | 审批组件可配置信息 | ApprovalConfig | {} |
openIntl | 是否开启多语言文案展示 | Boolean | false |
showAlias | 多语言文案渲染函数 | Function | - |
Events
方法名 | 描述 | 参数 |
---|---|---|
submit | 审批提交操作回调(通过、驳回、沟通等) | (data: FormData) |
Slot
插槽名 | 描述 | 参数 |
---|---|---|
content | 流程表单内容区域插槽 | - |
flow-diagram | 流程图区域插槽 | - |
Type
javascript
// 基本信息
interface Data{
title: string; // 标题
createTime: string; // 发起时间
creatorName: string; // 发起人
creatorDept: string; // 发起人部门
status: Status; // 状态,
flowToLabel: string; // 即将流向
currentResolver: string; // 当前处理人
}
// 流程状态
interface Status {
textColor:string;// 文本颜色
color: string; // 颜色
label: string; // 文案
}
// 操作记录
interface OperateRecord{
dateTime: string, // 操作时间
nodeName: string, // 节点名称
operator: string, // 操作人
operation: string,// 操作
comments: string, // 意见
files: Array<FileItem> // 附件列表
}
// 操作记录文件
interface FileItem {
uid: string, // 文件ID
name: string, // 文件名
url: string, // 文件URL
}
// 传阅记录
interface CirculateRecord{
dateTime: string; // 时间
sponsor: string; // 发起人
receiver: string; // 传阅对象
content: string; // 传阅内容
}
// 操作、选项、按钮
interface OptionItem {
value: string; // 键值
label: string; // 名称
}
// 审批组件额外配置
interface ApprovalConfig {
rejectConfig: RejectConfig; // 驳回配置
uploadConfig: UploadConfig; // 上传配置
userSelectConfig: UserSelectConfig; // 用户选择配置
operationConfig:OperationConfig; // 操作栏相关配置
}
// 驳回配置
interface RejectConfig {
rejectToList?: Array<OptionItem>; // 驳回到节点列表
rejectStrategyList?: Array<OptionItem>; // 驳回策略列表
}
// 上传配置
interface UploadConfig {
uploadCallback: Function
}
// 用户选择配置
interface UserSelectConfig {
api: string; // API
searchApi: string; // 搜索API
}
// 操作栏相关配置
interface OperationConfig {
commentLength: number; // 处理意见长度
showCommentWordLimit: boolean; // 是否显示处理意见字数限制
}
interface FormData{
operation: string; // 操作类型
delegate: Array<any>, // 转办人员
connector: Array<any>, // 沟通人员
rejectInfo: {
rejectActivityDefId: string; // 驳回目标活动定义ID
}
rejectStrategy: string, // 驳回策略
comment: string, // 意见
files: Array<FileItem> // 文件列表
}
interface FileItem {
file:File; // 文件
fileId:string; // 文件ID
name:string; // 文件名
}
使用示例
html
<template>
<van-loading size="24px" vertical v-if="pageLoading" class="page-loading"
>加载中...</van-loading
>
<HiFlowApproval
v-else
:data="taskInfo"
:operateRecords="operateRecords"
:circulateRecords="circulateRecords"
:operations="operations"
:approval-config="approvalConfig"
:open-intl="true"
:show-alias="showAlias"
@submit="submit"
>
<template #content>
流程表单组件
</template>
<template #flow-diagram>
流程图组件
</template>
</HiFlowApproval>
</template>
<script lang="ts" setup>
import HiFlowApproval from "@/lib/hi-flow-approval/index.vue";
import { ref } from "vue";
const workItemId = ref(1);
// 状态格式化方法
const formatterStatus = function (val) {
if (val == 1) {
return {
color: "grey",
label: "未启动",
};
} else if (val == 2) {
return {
color: "blue",
label: "运行",
};
} else if (val == 3) {
return {
color: "grey",
label: "挂起",
};
} else if (val == 7) {
return {
color: "green",
label: "完成",
};
} else if (val == 8) {
return {
color: "red",
label: "终止",
};
}
};
const data = ref({
creatorName: "测试",
creatorDept: "集团公司/流程IT与数据管理部/基础架构与云服务管理部",
createTime: "20240723175644",
status: formatterStatus(2),
title: "发起的流程图请审批",
flowToLabel: "部门长审批:张益达、吴琼",
currentResolver: "测试",
});
const operations = [
{
value: "save-submit",
label: "同意",
},
{
value: "reject",
label: "驳回",
},
{
value: "delegate",
label: "转办",
},
{
value: "connect",
label: "沟通",
},
{
value: "replyconnect",
label: "回复",
},
{
value: "cancelconnect",
label: "撤回沟通",
},
];
// 审批模板其他配置信息
const approvalConfig = ref({
rejectConfig: {
rejectToList: [
{
value: "code1",
label: "驳回到节点1",
},
],
rejectStrategyList: [
{
label: "按顺序流转",
value: "sequence",
},
{
label: "返回这个节点所有人",
value: "returnowner",
},
],
},
uploadConfig: {
uploadCallBack: fileUpload,
},
userSelectConfig: {
api: "",
searchApi: "",
},
});
// 操作记录
const operateRecords = ref([
{
dateTime: "2024-07-25 18:39:45",
nodeName: "发起",
operator: "测试",
operation: "发起",
comments: "发起流程1",
files: [
{
uid: "930051923594182656",
name: "config.txt",
url: "http://pangea-platform.clouddev.hisense.com/oss/files/pangea-test-bucket/flow/pangea/test.txt",
},
],
},
]);
// 传阅记录
const circulateRecords = ref([
{
dateTime: "2024-07-25 18:39:45",
sponsor: "测试",
receiver: "测试",
content: "发起流程1",
},
]);
// 提交
const submit = function (data) {
console.log(data);
};
onLoad((params: any) => {
// 获取页面参数
workItemId.value = 123456;
});
</script>
<style lang="less" scoped>
.page-loading {
height: 100vh;
justify-content: center;
}
</style>