流程图
流程图组件常用于审批流程设计场景,可对流程图的节点进行添加、删除、配置等操作。
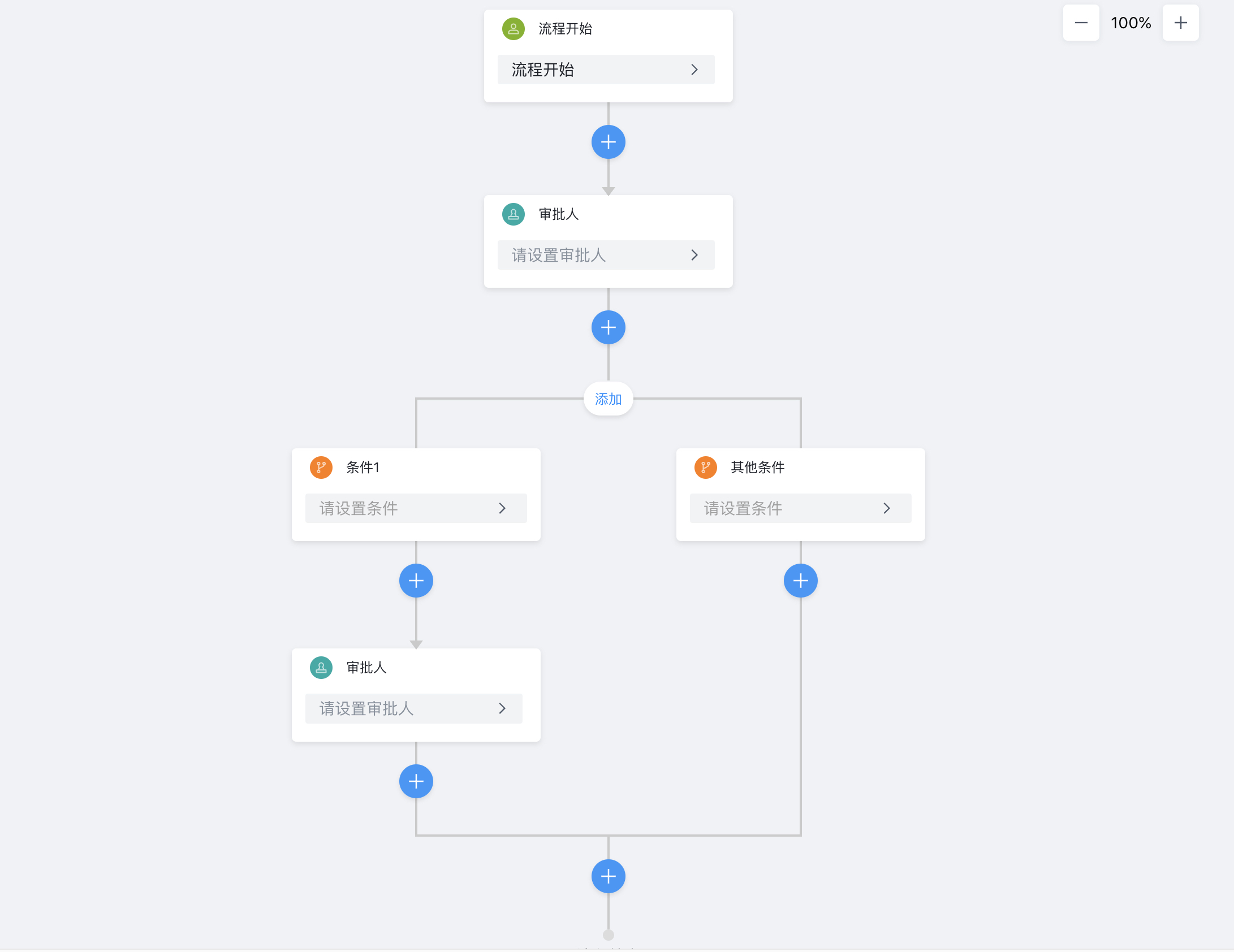
安装方式
bash
yarn add pangea-workflow
引用方式
js
import HiWorkflow from "pangea-workflow";
import "pangea-workflow/dist/style.css";
API
Props
参数名 | 描述 | 类型 | 默认值 |
---|---|---|---|
nodes | 流程图描述数据 | IRowNode | |
nodeTypeList | 节点类型列表 | INodeType | [] |
activeNode | 选中节点数据 | IRowNode | {} |
hideOperator | 是否隐藏操作类型按钮 | boolean | false |
hideFixedOperator | 是否隐藏悬浮操作 | boolean | - |
nodeProcess | 流程节点状态参数 | NodeProcess | {} |
extendConfig | 扩展配置 | ExtendConfig | {} |
Events
方法名 | 描述 | 参数 |
---|---|---|
clickNode | 点击流程节点时触发 | 返回当前节点数据(TreeNode) |
clickBlank | 点击空白位置时触发 | |
error | 异常时触发 | 返回异常相关信息(IFlowError) |
Methods
方法名 | 描述 | 参数 | 返回值 |
---|---|---|---|
getJson | 获取流程图数据 |
javascript
interface IRowNode {
data: Object; // 节点数据
childNode: Object; // 子节点
content: string; // 节点内容
id: string; // 唯一标识
type: string; // 节点类型
title: string; // 节点标题
nextIds: string; // 下一个节点的id
preIds: string; // 父节点id
}
interface INodeType {
data: Object; // 节点数据
color: string; // 图标背景颜色
icon: string; // 图标
category: string; // 分组标题
type: string; // 节点类型
value: string; // 节点标题
maxCount: number; // 节点最大数量
}
interface IFlowError {
type: string; // 异常类型 addError
data: INodeType | IRowNode; // 节点配置或节点信息
}
interface NodeProcess {
finishedNodes: Array<string>; // 完成节点id列表
activityNodes: Array<string>; // 活动节点id列表
pendingNodes: Array<string>; // 挂起节点id列表
terminatedNodes: Array<string>; // 终止节点id列表
}
interface ExpandOption {
label: string; // 选项文案
value: string; // 选项值
onClick: (value:string,node:IRowNode) => void; // 扩展选项点击回调函数
}
interface ExtendConfig {
routeExpandOptions: Array<ExpandOption> // 分支节点扩展选项
}
使用示例
html
<template>
<a-button @click="getData">获取数据</a-button>
<a-button style="margin-left: 8px" @click="setNodeData"
>修改节点数据</a-button
>
<HiWorkflow
ref="drawFlow"
:nodes="nodes"
:node-type-list="nodeOptions"
:hide-operator="false"
:extend-config="extendConfig"
:active-node="currentNode"
@click-node="clickNode"
@click-blank="clickBlank"
/>
</template>
<script lang="ts" setup>
import { ref, Ref } from "vue";
import HiWorkflow from "pangea-workflow";
import "pangea-workflow/dist/style.css";
// 流程图数据
const nodes = ref({}) as Ref;
const currentNode = ref({});
const drawFlow = ref();
const nodeOptions = [
{
type: "approval",
value: "审批人",
color: "#15bc83",
icon: "icon-user",
data: {},
},
{
type: "condition",
value: "条件分支",
color: "#ff943e",
icon: "icon-branch",
data: {},
},
];
// 扩展配置
const extendConfig = {
routeExpandOptions: [{
value:"config",
label:"分支配置",
onClick: (value: string, node: any)=>{
console.log("分支配置", value, node)
}
}]
}
// 节点点击
const clickNode = (node: any, e: any) => {
console.log("节点数据", node);
currentNode.value = node;
};
// 空白点击
const clickBlank = (e: any) => {
console.log("空白位置", e);
};
// 获取流程图数据
const getData = () => {
console.log("流程图数据", drawFlow.value.getNodes());
};
// 修改节点数据
const setNodeData = () => {
// 修改当前激活节点内容
currentNode.value.content = "张益达";
};
</script>